We all have learned sorting techniques in our college academic curriculum. Out of those sorting techniques, the simplest technique we learned is Bubble Sort in Java. The Bubble sort takes an array of numbers and sorts them within the array indexes in either ascending or descending order. Bubble sort is the simplest of all sorting techniques but it not efficient as other sorting methods.
Bubble Sort in Java
In Bubble sort the smallest or largest number, based on whether you want to sort ascending or descending order, it bubbles up the number towards the start or end of the array. We will need “N” numbers of iterations through the array, where “N” is the length of the array, to sort an array of numbers. At the end of each iteration, one number is placed in its proper order.
First, you need to do in Bubble Sort is to create an array of numbers. You can create an array of 2 numbers or thousands of numbers and can start sorting by taking the first number in the array and comparing it with the number adjacent to it and start swapping if the number is less than or greater than based on the order of sorting i.e ascending or descending. You can either start comparing from the start or end of the array. In our example, we are comparing from the start of the element.
The below picture shows your first iteration of sorting an element in the array. As it is always said picture speaks more than a thousand words.
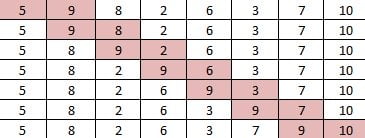
Java code to Bubble sort in ascending and descending order
/****************************************************************************************
* Created on Sept 15, 2014 Copyright(c) https://kodehelp.com All Rights Reserved.
****************************************************************************************/
package com.kodehelp.java;
import java.lang.reflect.Array;
import java.util.Arrays;
/**
* Created by https://kodehelp.com
*/
public class BubbleSortExample {
public static void main(String args[]) {
int[] numbers = { 5, 9, 8, 2, 6, 3, 7, 10 };
print(numbers);
int[] ascNumbers = sortAscending(numbers);
print(ascNumbers);
int[] dscNumbers = sortDescending(numbers);
print(dscNumbers);
}
public static void print(int[] numbers) {
for (int i : numbers) {
System.out.print(i + " ");
}
System.out.println(" ");
}
public static int[] sortAscending(int[] numbers) {
int temp;
for (int i = 0; i < numbers.length; i++) {
for (int j = 0; j < numbers.length - 1; j++) {
if (numbers[j] > numbers[j + 1]) {
temp = numbers[j + 1];
numbers[j + 1] = numbers[j];
numbers[j] = temp;
}
}
}
return numbers;
}
public static int[] sortDescending(int[] numbers) {
int temp;
for (int i = 0; i < numbers.length; i++) {
for (int j = 0; j < numbers.length - 1; j++) {
if (numbers[j] < numbers[j + 1]) {
temp = numbers[j + 1];
numbers[j + 1] = numbers[j];
numbers[j] = temp;
}
}
}
return numbers;
}
}