To start with any tutorial is to start Hello World Example. In this tutorial we will see JSF 2.0 Hello World Example below
Download Source code for tutorial
Download here – JavaServerFaces 2.0 – Hello World Example – Source code
Setup Development Environment For JSF 2.0 Hello World Example
To start with JavaServer Faces Hello World Example, you need to setup development environment. Below list shows you, how to setup tutorial’s development environment, dependencies and basic configurations.
Creating JavaServerFaces Project in Eclipse
Step 1 : Create New Dynamic Web Project
- Create New Dynamic Web Project by clicking File àNewà Dynamic Web Project and give project name as “JavaServerFaces”.
- Select the target runtime. If there is no target runtime already created, click on New Runtime button and create the target runtime. This is the target server where your application will be running.
- Select Dynamic Web module version as 3.0
- Select Configuration as JavaServer Faces v2.0 Project
- Click Next
Please see below screenshot for step 1.
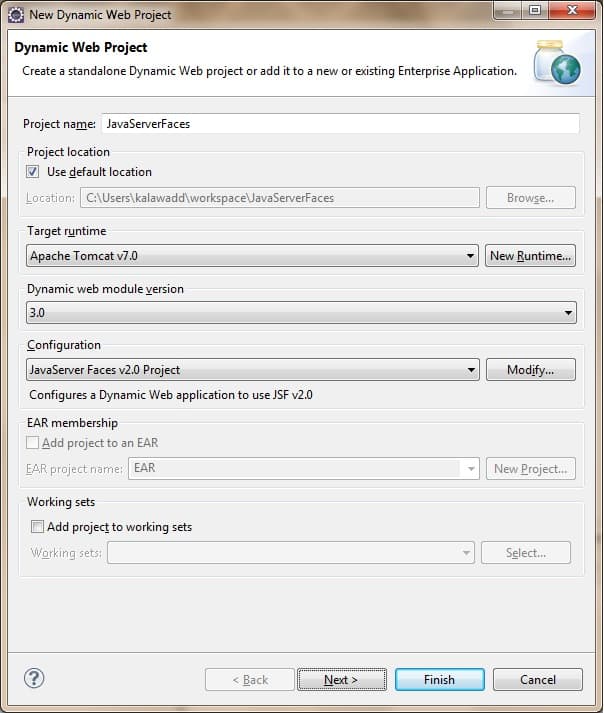
Step 2 : Configure project for building a Java application.
- Select Source ( src) and output folder as show in below screenshot.

Step 3 : Configure web module settings.
- Select default values for context root and context directory.
- Select Generate web.xml deployment descriptor checkbox.
- Click on the finish button to create the JSF Project in the eclipse workspace.

Final project structure, in case you get lost or confused about the creation of project files –

JSF 2.0 Servlet Configuration in web.xml
To run the JSF 2.0 application, you need to configure the JSF servlet in web.xml, similar to any other web framework.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name>JavaServer Faces 2.0 Hello World Application</display-name>
<!– Change to "Production" when you are ready to deploy –>
<context-param>
<param-name>javax.faces.PROJECT_STAGE</param-name>
<param-value>Development</param-value>
</context-param>
<!– Welcome page list –>
<welcome-file-list>
<welcome-file>index.xhtml</welcome-file>
</welcome-file-list>
<!– JavaServer Faces Servlet –>
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<!– Servlet Mapping to URL pattern –>
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.jsf</url-pattern>
</servlet-mapping>
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.faces</url-pattern>
</servlet-mapping>
</web-app>
Add “javax.faces.webapp.FacesServlet” servlet and map it to different URL patterns as below –
- *.xhtml
- *.jsf
- *.faces
In JSF 2.0 development, it’s recommended to set the “javax.faces.PROJECT_STAGE” to “Development“, it will provide many useful debugging information to let you track the bugs easily. For deployment, just change it to “Production“.
JSF 2.0 Managed Bean
Managed bean is nothing but a regular java bean which has received as fancy name in JSF terminology. Whenever a bean is registered with the JavaServer Faces it becomes a managed bean. Managed beans are used as a model for UI components. Prior to JSF 2.0, managed bean was registered in faces-config.xml but now in JSF 2.0, there is no need to declare managed bean in the config xml file. Instead in JSF 2.0 managed beans are registered using @managedbean annotation.
Create a managed bean in package com.kodehelp.jsf as below. You can prefer any package name.
package com.kodehelp.jsf;
import java.io.Serializable;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean
@RequestScoped
public class HelloWorldBean implements Serializable {
private static final long serialVersionUID = 1L;
private String firstName;
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
JSF 2.0 UI Pages
The standard for creating the UI pages is in .xhtml format and this is recommended in JSF.
I will be writing on UI Tags soon. Please keep watching https://kodehelp.com
To use JSF tags in UI page you need to include below namespaces in the html tag –
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" xmlns:h="http://java.sun.com/jsf/html" xmlns:f="http://java.sun.com/jsf/core">
To use application.properties file you need to add below JSF tag –
<f:loadBundle basename="resources.application" var="msg"/>
And you a can use as the application.properties as shown below –
# -- welcome --
welcomeTitle=JSF Hello World Application
welcomeHeading=Welcome!
welcomeMessage=This is a JSF Hello World application. \
Please Enter your First Name and Last Name below.
helloHeading=Hello!
helloMessage=This is a JSF Hello World application. \
Enjoy your JSF Learning and there is much more to learn in JSF 2.0. \
Please stay with Vigilance series of JSF 2.0 Tutorial at
<head>
<title><h:outputText value="#{msg.welcomeTitle}" /></title>
</head>
File: index.xhtml –
- Renders 2 outputText which shows the property value from application.properties file.
- Renders 2 outputLabel for First Name and Last Name.
- Renders 2 textbox for firstName and lastName which are attached with respective managed bean property.
- Renders a button which displays hello.xhtml
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" xmlns:h="http://java.sun.com/jsf/html" xmlns:f="http://java.sun.com/jsf/core">
<f:loadBundle basename="resources.application" var="msg" />
<head>
<title>
<h:outputText value="#{msg.welcomeTitle}" />
</title>
</head>
<body>
<h3>
<h:outputText value="#{msg.welcomeHeading}" />
</h3>
<h:outputText value="#{msg.welcomeMessage}" />
<h:form>
<h:panelGrid columns="2">
<h:outputLabel for="firstName">First Name *</h:outputLabel>
<h:inputText id="firstName" value="#{helloWorldBean.firstName}"></h:inputText>
<h:outputLabel for="lastName">Last Name *</h:outputLabel>
<h:inputText id="lastName" value="#{helloWorldBean.lastName}"></h:inputText>
<h:commandButton value="Submit" action="hello"></h:commandButton>
</h:panelGrid>
</h:form>
</body>
</html>
File: hello.xhtml – This page renders the value from the managed bean for First name and Last Name entered on the index.xhtml.
<?xml version="1.0" encoding="ISO-8859-1"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:f="http://java.sun.com/jsf/core" xmlns:h="http://java.sun.com/jsf/html">
<f:loadBundle basename="resources.application" var="msg" />
<head>
<title>
<h:outputText value="#{msg.welcomeTitle}" />
</title>
</head>
<h:body>
<h3>
<h:outputText value="#{msg.helloHeading}" />
#{helloWorldBean.firstName} #{helloWorldBean.lastName}
</h3>
<h:outputText value="#{msg.helloMessage}" />
<a href="http://vigilance.o.in">Vigilance.co.in</a>
</h:body>
</html>
The #{ } indicate as a JSF expression language, in this case, #{helloWorldBean.firstName} and #{helloWorldBean.lastName}, when the page is submitted, JSF will find the “helloWorldBean” and set the submitted textbox value via the setFirstName() method. When hello.xhtml page is displayed, JSF will find the “helloWorldBean” and displays the firstName and lastName property value via the getFirstName() and getLastName() methods respectively.
Running the JSF 2.0 Hello World Application
To run this application from eclipse, right click on the project à Run As à Run On Server
Select the tomcat 7.0 server and run the application.
Go to url http://localhost:8080/JavaServerFaces/ for view the page.
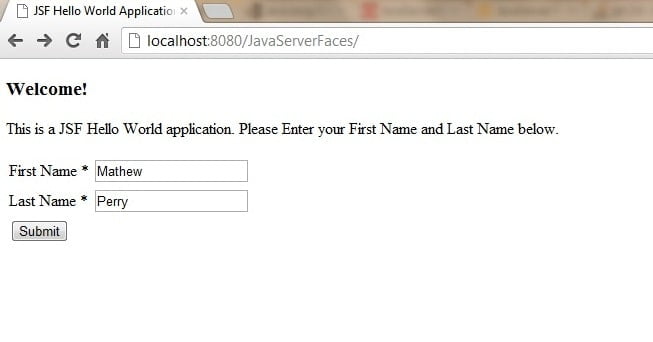