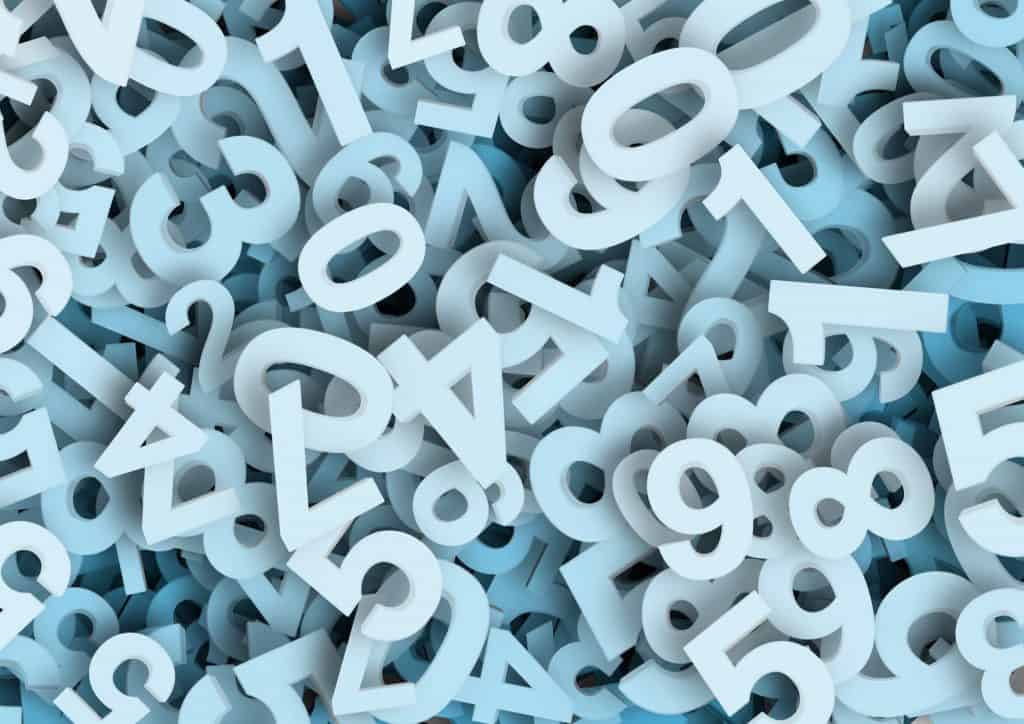
In this post, we will see how to use the Javascript Random Number Generator. Math.random() function is used to generate random number(s) in Javascript. The most common use cases for generating random numbers are games of chance like rolling dice, shuffling playing cards, and spinning roulette wheels.
Random numbers are the numbers that occur in sequence such that the values are uniformly distributes over a defined interval or set, which is impossible to predict future values based on present and past values. Also, it should pass a bunch of statistical tests. Random Numbers are important in statistical analysis and probability theory.
This article shows you different approaches for generating a random number in Javascript –
1. Javascript Random Number Generator with Math.random()
The Math object in JavaScript is a built-in object that has properties and functions for performing mathematical calculations. A very common use of the javascript Math object is to create a random number using the random() function.
Math.random() function does not actually return a whole number. Its return a floating point, pseudo-random number in the range between 0 (inclusive) and 1 (exclusive).
Random numbers generated by Math.random() are not actually random. It might seem random, but those numbers will repeat and eventually display a non-random pattern over a period of time.
for(var i=0; i<5; i++){
const randomValue = Math.random();
console.log(randomValue);
}
Output
0.6886825676948469
0.3628905443572823
0.3077570956133415
0.3240039650675881
0.49029283306138205
Math.random()
does not provide cryptographically secure random numbers. Do not use them for anything related to security. Use the Web Crypto API instead.
2. Generate Random Numbers between 2 values
Now, let’s create a function that uses Math.random() and returns a random number between two values.
const getRandomNumber = (min, max) => {
return Math.random() * (max - min) + min;
};
for(var i=0; i<5; i++) {
console.log(getRandomNumber(2,3));
}
Output
2.625680183263521
2.961340340002487
2.3652621562197007
2.112806882467397
2.9610930343245245
The above code will return a floating-point value between 2 (inclusive) and 3 (exclusive).
3. Generate Random Integers between 2 values
This example returns a random integer between the specified values min (inclusive) and max (exclusive)
const getRandomNumber = (min, max) => {
min = Math.ceil(min);
max = Math.floor(max);
//The maximum is exclusive and the minimum is inclusive
return Math.floor(Math.random() * (max - min) + min);
};
for(var i=0; i<5; i++) {
console.log(getRandomNumber(1,30));
}
Output
8
14
2
19
24
The above code returns an integer value between 2 (inclusive) and 30 (exclusive).
4. Generate Random Integers between 2 values (Inclusive)
This example returns a random integer between the specified values min (inclusive) and max (inclusive)
const getRandomNumber = (min, max) => {
min = Math.ceil(min);
max = Math.floor(max);
//The maximum is exclusive and the minimum is inclusive
return Math.floor(Math.random() * (max - min+1) + min);
};
for(var i=0; i<5; i++) {
console.log(getRandomNumber(1,6));
}
Output
1
4
3
2
6
The above code returns an integer value between 1 (inclusive) and 6 (inclusive).