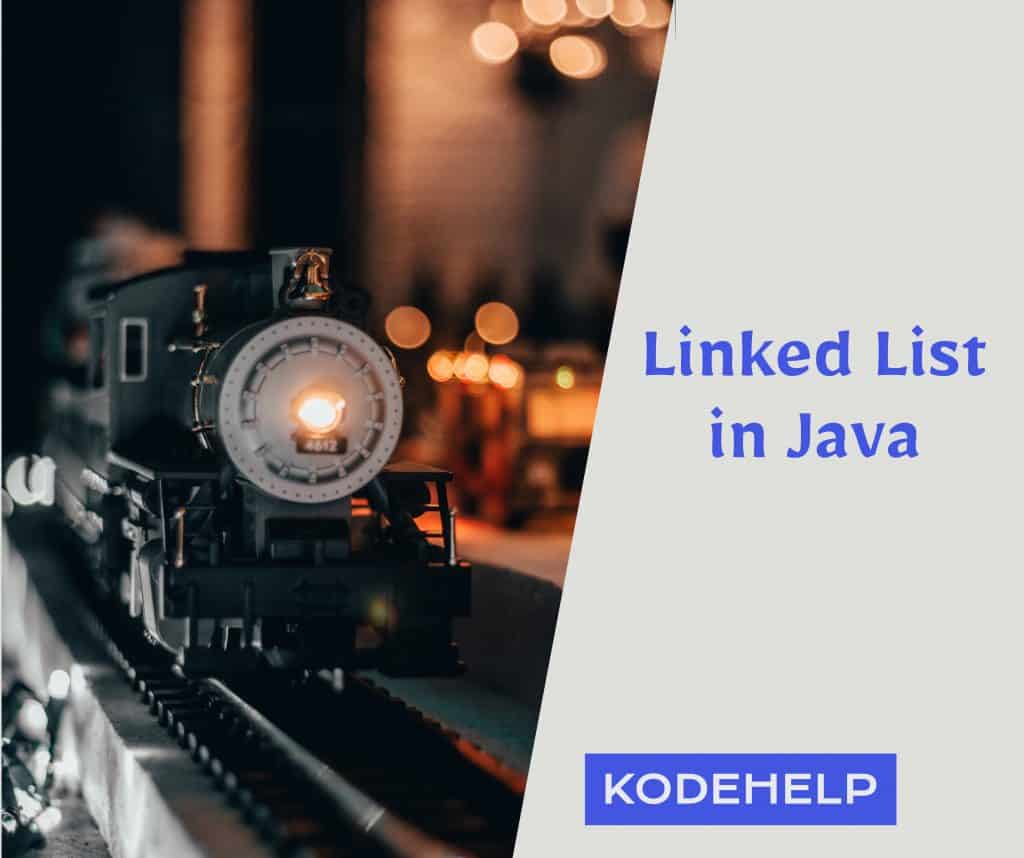
Linked List in Java is a Collection class and it implements the doubly linked list data structure. LinkedList class in Java extends an abstract class AbstractSequentialList and implements the List and Deque interfaces. It implements all optional list operations and permits all elements, including null
.
For those, who are not familiar with a linked list data structure, it is a linear data structure that does not store the elements in contiguous locations and every element is a separate object with a data part and address part. In Linked list elements uses pointers and addresses to link each other. Each element in a linked list is known as a node. The benefits of using a linked list over Array are its simplicity and ease of insertions and deletions. The disadvantage of using the linked list is that it cannot be accessed directly. Instead, we need to start from the head and follow through the link to reach a node we wish to access.
There is two kinds of linked list, singly and doubly linked list. A singly-linked list allows traversing in one direction, mostly forward direction, whereas a doubly linked list allows to traverse in both directions, forward, and backward.
1. Create and Use Linked List in Java
The following implementation demonstrates how to create and use a linked list in Java.
package com.kodehelp.java.collection;
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
// Creating object of the class linked list
LinkedList<String> linkedList = new LinkedList<>();
// Adding elements to the linked list
linkedList.add("Java");
linkedList.add("Kotlin");
linkedList.addLast("Python");
linkedList.addFirst("Scala");
linkedList.add("Dart");
linkedList.add(1, "Go");
System.out.println(linkedList);
linkedList.removeFirst();
System.out.println("After removeFirst() : "+linkedList);
linkedList.removeLast();
System.out.println("After removeLast() : "+linkedList);
linkedList.remove("Kotlin");
System.out.println("After remove(\"Kotlin\") : "+linkedList);
linkedList.remove(2);
System.out.println("After remove(index) : "+linkedList);
}
}
OUTPUT
[Scala, Go, Java, Kotlin, Python, Dart]
After removeFirst() : [Go, Java, Kotlin, Python, Dart]
After removeLast() : [Go, Java, Kotlin, Python]
After remove("Kotlin") : [Go, Java, Python]
After remove(index) : [Go, Java]
2. Java LinkedList Operations
Below we will see how to perform some basics operations on LinkedList –
2.1 Adding Elements to LinkedList
To add an element to a LinkedList, we can use the add() method. This method is overloaded to perform multiple operations based on different parameters. These methods to add elements are as follows –
- add(Element E): This method inserts an element at the end of the LinkedList.
- addFirst(Element E): This method inserts the specified element at the beginning of this list.
- addLast(Element E): This method inserts the specified element at the end of this list.
- add(int index, Element E): This method inserts an element at a specific index in the LinkedList.
Following Java code shows how to use add elements to a Linked List –
package com.kodehelp.java.collection;
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
// Creating object of the class linked list
LinkedList<String> linkedList = new LinkedList<>();
// Adding elements to the linked list
System.out.println("Before adding Elements : "+linkedList);
linkedList.add("Java");
linkedList.add("Kotlin");
linkedList.add("Dart");
System.out.println("Before adding Element using addLast(E) : "+linkedList);
linkedList.addLast("Python");
System.out.println("Before adding Element using addFirst(E) : "+linkedList);
linkedList.addFirst("Scala");
System.out.println(linkedList);
}
}
OUTPUT
Before adding Elements : []
Before adding Element using addLast(E) : [Java, Kotlin, Dart]
Before adding Element using addFirst(E) : [Java, Kotlin, Dart, Python]
[Scala, Java, Kotlin, Dart, Python]
2.2 Replacing or Changing an Elements in LinkedList
After adding the elements to Linked List, if you wish to change the element, it can be done using the set(int index, Element E) method. Following example shows how to replace an element in a linked list –
package com.kodehelp.java.collection;
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
// Creating object of the class linked list
LinkedList<String> linkedList = new LinkedList<>();
// Adding elements to the linked list
linkedList.add("Java");
linkedList.add("Kotlin");
linkedList.add("Dart");
System.out.println("Elements in LinkedList: "+linkedList);
linkedList.set(2, "Python");
System.out.println("After replacing an element in Linked List : "+linkedList);
}
}
OUTPUT
Elements in LinkedList: [Java, Kotlin, Dart]
After replacing an element in Linked List : [Java, Kotlin, Python]
2.3 Removing Elements from Linked List
In order to remove an element from a LinkedList, we can use the remove() method. There are different methods available to remove an element from Linked List. These methods are as follows –
- remove(Element): This method removes an element from the LinkedList. If there are multiple such objects, then it removes the first occurrence of the element.
- removeFirst(): This method removes and returns the first element from this list.
- removeLast(): Thiis method removes and returns the last element from the list
- remove(int index): This method takes an integer value and simply removes the element present at that specific index in the LinkedList. After removing the element, all the elements move to the left to fill the space and the indices of the elements are updated.
Following Java code shows how to use remove elements from a Linked List –
package com.kodehelp.java.collection;
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
// Creating object of the class linked list
LinkedList<String> linkedList = new LinkedList<>();
// Adding elements to the linked list
linkedList.add("Java");
linkedList.add("Kotlin");
linkedList.add("Dart");
linkedList.add("Python");
linkedList.add("Scala");
System.out.println("Before removing elements :"+linkedList);
linkedList.remove(2);
System.out.println("After remove(index) : "+linkedList);
linkedList.removeFirst();
System.out.println("After removeFirst() : "+linkedList);
linkedList.removeLast();
System.out.println("After removeLast() : "+linkedList);
linkedList.remove("Kotlin");
System.out.println("After remove(\"Kotlin\") : "+linkedList);
}
}
OUTPUT
Before removing elements :[Java, Kotlin, Dart, Python, Scala]
After remove(index) : [Java, Kotlin, Python, Scala]
After removeFirst() : [Kotlin, Python, Scala]
After removeLast() : [Kotlin, Python]
After remove("Kotlin") : [Python]
Hope above details and examples help you to clarify your Java Linked List concepts.
If you like this post, please share among your friends.