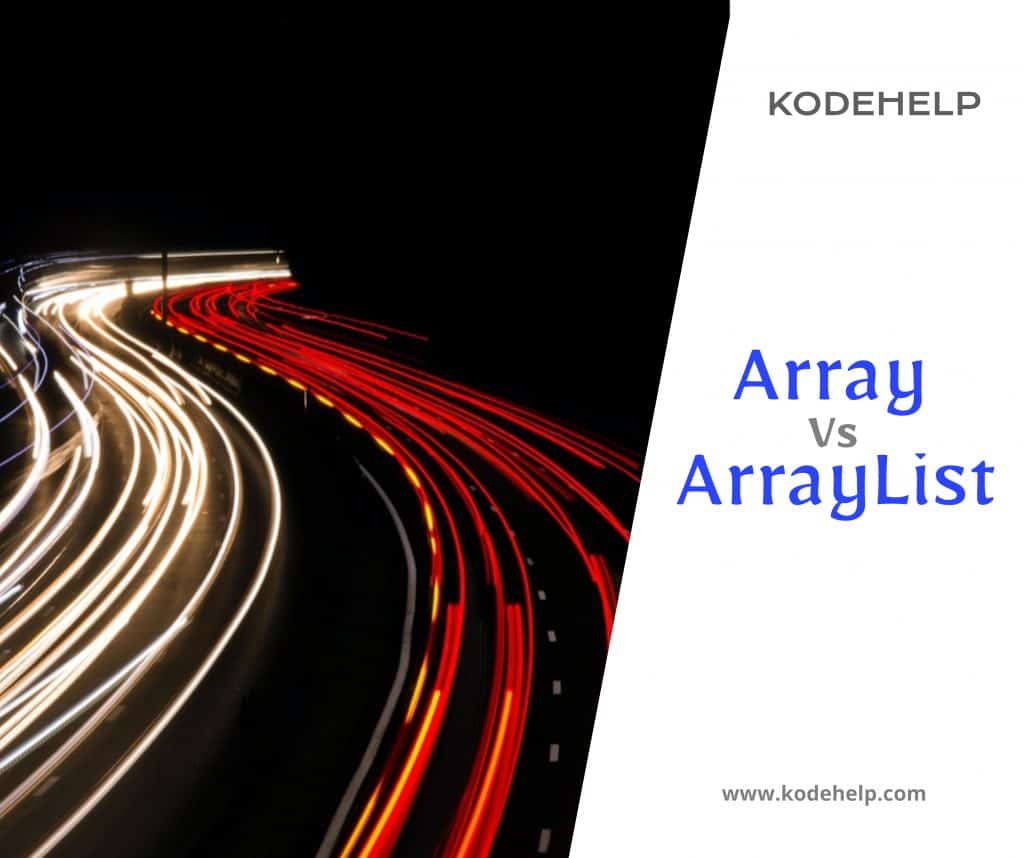
Both Array and ArrayList are very important and frequently used data structures in Java. The main difference between Array Vs ArrayList is that Array is a fixed-length data structure whereas ArrayList is a variable-length Collection class in Java. Even though Array backs an ArrayList, knowing the difference between an array and an ArrayList in Java is critical for becoming a good Java developer. Knowing this difference is also a very common question among beginners especially those who started coding in Java.
This article will help you understand the difference between ArrayList Vs Array in Java. If you are coming from another programming language like C or C++ background then you already know that array is one of the most useful data structures in the programming world.
Below are the parameters that we will discuss the difference between Array and ArrayList –
1. Nature of Array Vs ArrayList
An array is static in nature, which means it is a fixed-length data structure. Once we declare the length at the time of array creation, we cannot change its size again.
int arr[]=new int[7]; //specified size of array is 7 and cannot be change
ArrayList is dynamic in nature, the size of the ArrayList can be changed once it is created. The default size of ArrayList in java is 10. But an ArrayList is a growable array, unlike an array it doesn’t have a fixed length. It increases the size dynamically whenever we add or remove any element in ArrayList. We can initialize the capacity of ArrayList during the creation of ArrayList. The ArrayList class provides a constructor that takes initial capacity as an integer value.
ArrayList<Integer> list = new ArrayList<Integer>(15);
2. Implementation
The array is a native programming component or data structure whereas ArrayList is a class from the Java Collections framework. ArrayList in Java is internally implemented using Arrays. Since ArrayList is a class it holds all properties of a class e.g. you can create objects and call methods but even though the array is an object in Java it doesn’t provide any method. It just exposes a length attribute to give you the length of the array, which is constant.
3. Performance
Since ArrayList internally works based on the array, you might think that performance of ArrayList is the same as Array. This is actually true to some extent but the performance of ArrayList may be slower as compared to Array because it has some extra functionality other than Array. The performance impact for ArrayList is mainly in terms of CPU time and memory usage.
Index-based access of elements for both Array and ArrayList provides the same performance which is O(1)
whereas add operation in ArrayList can be expensive as O(logN)
. Adding a new element in ArrayList triggers resize, as it involves creating a new array in the background and copying elements from the old array to the new array.
ArrayList requires more memory than an array for storing the same number of objects. For example, an int[]
will take less memory to store 30 int
variables than an ArrayList because of object metadata overhead on both ArrayList and wrapper class.
4. Type of data stored in Array and ArrayList
The array can hold both primitive data types or objects of a class as the array elements. ArrayList can store only objects and cannot allow storage of the primitive types. This is a key difference between array and ArrayList. Whenever you pass an int value to the add method of ArrayList, it converts that number into the Wrapper class Integer object. Thus, we can say that Autoboxing from Java 5 onwards provides you the way to use the primitive data types in the ArrayList.
For Example :
ArrayList arrayList = new ArrayList();
arrayList.add(30); // add 30 int primitive data type value
JVM automatically converts the primitive data type value into the equivalent object. It ensures that only objects are added to the ArrayList as shown below –
// Converting int primitive value to Integer object and added to arrayList object.
arrayList.add(new Integer(30));
5. Type Safety
One more difference between Array and ArrayList is that ArrayList is type-safe because it supports the Generics which allows the compiler to check if all objects stored in ArrayList are of the correct type. On the other hand, the array doesn’t support Generics in Java. This means compile-time checking is not possible but array provides runtime type checking by throwing ArrayStoreException
if you try to store an incorrect object into an array. For example: Storing a String into an int array.
6. Flexibility of Array Vs ArrayList
Flexibility is an important factor that differentiates the Array and ArrayList in Java. ArrayList is more flexible than a plain native array because it is dynamic. When you add elements beyond ArrayList’s capacity, it can grow automatically. ArrayList also allows removing elements from it while it is not possible with arrays. We can’t remove elements from an array after adding them. You can learn more about removing objects from ArrayList in my article How to Remove Element from ArrayList in Java?
7. Iteration of Array and ArrayList
You can iterate Array only with the help of for loop, for-each, or a while loop. Arrays do not support an iterator to iterate it as the case in ArrayList. On the other side, to iterate ArrayList you have many different ways. You can use the Iterator and ListIterator class to iterate over an ArrayList. However, you can also use the for, while, and for-each loops to iterate an ArrayList.
8. Dimension
Another important difference between Array Vs ArrayList is that the array can be multi-dimensional. You can create a two-dimensional array or a three-dimensional array, which makes it a really special data structure to represent matrices, 2D, and 3D data. On the other side, ArrayList cannot be multi-dimensional, it can only be one or single-dimensional. In fact, it does not allow you to specify the dimension. It is by default one-dimensional in nature.
A multidimensional array is an array of arrays. Each element of a multidimensional array is an array itself. For example,
int[][] a = new int[3][4];
9. Size() vs length
As Array is an object and has a single attribute called length and that too is constant. This length attribute tells you the number of slots in the array i.e. how many elements it can store, it doesn’t provide you any method to find out how many are filled and how many slots are empty i.e. the current number of elements.
On the other hand, ArrayList does provide a size() method which tells the number of objects stored in ArrayList at a given point of time. The size() is always different than the length, which is also the capacity of ArrayList.
Example :
//using length attribute to find the length of Array
int array[] = new int[7];
arrayLength = array.length;
// using Size() method to find the size of an ArrayList
ArrayList arrayList = new ArrayList();
arrayList.add(17);
arrayListt.size();
10. Supported Operations
Both ArrayList and array also provide ways to retrieve elements like get() method of ArrayList uses an index to get an element from array e.g. students[0] will return the first element. ArrayList also provides an operation to clear and reuse e.g. clear() and removeAll(), the array doesn’t provide that but you can loop over Array and assign each index null to simulate that.
In short, ArrayList supports methods such as get(), isEmpty(), indexOf(), replaceAll(), contains(), clear(), removeAll(). There is no support for such methods in Arrays.
Conclusion
Above points helps you understand the differences between Array Vs ArrayList. To use ArrayList you need to import the package java.util.ArrayList
, while to use array you need not import any packages in your class. ArrayList is more flexible than arrays as it comes with many inbuilt functionalities but at the same time, it has poor performance efficiency than arrays.